As modern web applications adopt increasingly complex architectures, comprehensive end-to-end testing, including Playwright testing, becomes critical to ensure seamless functionality. However, configuring the environment to run robust testing tools like Playwright can be tedious due to multiple dependencies. This is where Docker can streamline the process.
In this article, we will explore best practices for containerized testing and how Docker containers can optimize Playwright-based testing of complex modern web applications.
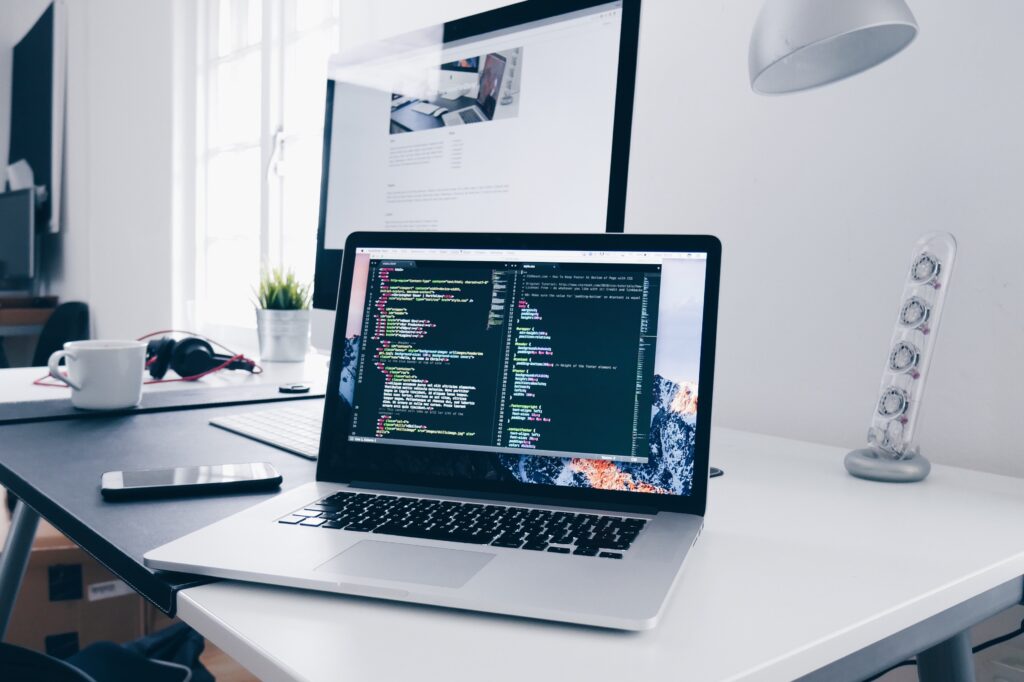
What is Docker?
Docker is an open platform that enables developers to easily pack, ship, and run applications within lightweight containers. Containers bundle all the code, frameworks, dependencies, and system libraries required for applications to function seamlessly across environments.
Unlike virtual machines, which run an entire guest operating system, Docker containers share the host system’s Linux kernel and only require libraries not already running on the host. This makes containers extremely portable and resource-efficient.
Developers can build Docker images, which serve as immutable templates for containers. Images can be shared via repositories like Docker Hub, so applications are reproducibly built and launched. Containers act as standardized building blocks, ensuring environment consistency across development, testing, and production.
By containerizing the entire application environment, Docker eliminates “works on my machine” bugs stemming from underlying infrastructure and dependency differences. With Docker, the software can move frictionlessly from a developer’s laptop to enterprise data centers without modification or performance impacts.
Overall, Docker empowers developers with a platform for building, distributing, and running portable applications to unlock velocity and reliability. Docker overcomes key pains in the development and deployment lifecycle by containerizing software.
Why Docker?
Docker helps developers and operations teams solve several major pain points in the software development and deployment process. By containerizing applications, Docker streamlines how code gets built, shipped, and run across different environments.
- Avoids Dependency and Configuration Issues: Docker containers include all the dependencies and configs an app needs to be baked into the container image. This provides predictable environments that avoid “works on my machine” problems when code moves through dev, test, and prod. The same container will run exactly the same way on any infrastructure.
- Enables Easy and Efficient Scaling: You can spin up multiple container replicas rapidly without provisioning entire new servers each time. This enables agile scaling and resource efficiency. Docker’s lightweight containers make scaling up and down simple.
- Provides Portability Across Environments: Docker images can be easily shared through registries like Docker Hub and run on any system or cloud with Docker installed. This simplifies deployment and distribution. Docker containers provide a standard, portable artifact that runs consistently on any infrastructure.
- Offers Fast Deployment and Near-Native Performance: Since containers share the host OS kernel, they start instantly and have near-native performance. This makes Docker ideal for microservices architectures where you want to break monoliths into lightweight distributed services.
- Provides Default Isolation and Security: Containers provide isolation and security by default. Each container shares only the dependencies it needs, limiting the attack surface. Resources are contained and accounted for rather than spread across servers.
- Streamlines Software Delivery Pipelines: Overall, Docker enables DevOps teams to ship software faster and more reliably. Standard containers provide a consistent way to develop, test, deploy, and scale applications across different infrastructures. This reduces bugs and speeds up delivery.
- Enables Focus on Code Instead of Environments: With Docker, developers can focus on writing code rather than battling environments and dependencies. Operations teams get reliable, scalable applications that deploy consistently across hybrid cloud environments.
In brief, Docker provides portability, consistency, and scalability – delivering faster software life cycles.
Docker Architecture
Docker consists of a client-server architecture, where the Docker client communicates with the Docker daemon to build, run, and manage containers.
Before we proceed with the installation and writing of tests, let’s first understand the Docker Architecture.
Docker Client:
- The primary user interface to Docker. It accepts commands from users and communicates with the Docker daemon.
- Available as a binary (docker) or via language-specific SDKs and APIs.
- Common commands: docker run, docker build, docker pull, docker push, etc.
Docker Daemon:
- A background service/process that runs on the host machine.
- Responsible for managing Docker objects like images, containers, networks, and volumes.
- Listens to API requests from clients and manages Docker services.
- Builds images from Dockerfiles and creates containers from images.
- Interacts with Containers to run containers.
Docker Host:
- The physical server or virtual machine where Docker is installed.
- Runs and manages Docker daemon and containers.
- Provides OS-level virtualization features like namespaces and cgroups.
- Can be a Linux or Windows system running locally or in the cloud.
Docker Registries:
- Used to store, distribute, and download Docker images.
- The default public registry is Docker Hub, with 100,000+ images.
- Users can deploy private registries to store images securely.
- Images are pushed and pulled from registries.
Docker Images:
- Read-only templates are used to create Docker containers.
- Created from Dockerfile build instructions.
- Stored in registries and pulled to hosts to create containers.
- Made up of layered filesystems with libraries, dependencies, and code.
Docker Containers:
- Running instances of Docker images.
- Offer isolated environments for applications.
- Created from images using docker run.
- Share host kernel, run as an isolated user process.
- Provide compute resources, networking, and storage to apps.
Docker Networking:
- Enables communication between containers, hosts, and external networks.
- Default bridges provide basic connectivity.
- Custom networks with scoping and driving policies can be created.
- Overlay networks connect multiple Docker daemons.
Docker Storage:
- Manages persistent data for containers via volumes bind mounts.
- Volumes are preferred over bind mounts.
- Storage drivers and plugins allow integration of storage systems.
- tmpfs mounts provide temporary, ephemeral storage.
OCI Standards:
- Open Container Initiative defines common container standards.
- Enables consistency and portability across platforms.
- Standards for container image format, runtime, and distribution.
- Adopted by Docker, Kubernetes, and cloud providers.
Docker provides a standardized platform for developing, shipping, and running containerized applications across diverse infrastructures. Key components include the Docker engine, images, containers, registries, and tools for networking and storage. OCI standards help enhance portability across Docker and other container platforms. Together, these capabilities simplify the deployment and scaling of applications across different environments.
Installing Docker
Install Docker on your machine if not already installed. On Linux, use your package manager. On Mac or Windows, download from https://www.docker.com/products/docker-desktop.
Verify Docker is installed:
docker –version |
Verify Docker service is running:
docker info |
Writing Playwright Tests
Create a new directory for the tests:
mkdir playwright-tests |
Initialize NPM project:
npm init -y |
Install Playwright as a dev dependency:
npm install -D @playwright/test |
Create a test file tests/example.spec.js
Add a sample test:
//js |
Run in Docker
Create a Dockerfile:
//dockerfile |
Build Docker image:
docker build -t playwright-tests . |
Run the image:
docker run -it –rm playwright-tests |
We will run the tests inside a Docker container using the image we built.
Best Practices for Containerized Testing with Docker
These comprehensive best practices will enable maximum reliability, portability, and optimal resource usage for container-based test automation.
- Use Small Base Images: Start with a minimal base image like Alpine Linux when building test containers. Alpine is a small distribution that only includes essential packages, keeping images extremely lean at less than 5MB. Other small base images are Debian Slim and Ubuntu Minimal. Avoid fat images like Ubuntu, which can be 100MB+.
- Cross-Browser Testing: Leverage platforms like LambdaTest that offer a scalable way to test browser compatibility across multiple browsers and operating systems in the cloud. Â LambdaTest is an AI-powered test orchestration and execution platform that lets you run manual and automated tests at scale with over 3000+ real devices, browsers, and OS combinations. This provides wide coverage without manually configuring a complex matrix of platforms.
- Leverage Multi-Stage Builds: Use multi-stage builds in your Dockerfile to optimize the final image. In the early stages, include tools for building dependencies like compilers, build systems, etc. In the final stage, copy only the necessary artifacts, like the test code, configs, and binaries. This keeps containers tidy and minimal.
- Isolate Test Environments: Maintain separate containers for each testing environment, such as development, staging, production, smoke testing, etc. Keeping environments isolated promotes proper replication and avoids interference across containers.
- Create Repeatable Builds: Bake dependencies, configurations, and setup directly into the Dockerfile rather than running them manually. This enables repeating consistent container builds that provide the exact same testing environment every time.
- Externalize Test Data: For portability, store test data like fixtures, templates, and expected results on mounted volumes rather than directly in the container. The test code can be rebuilt while the data persists on the host.
- Parallelize Test Execution: Â Use Docker Compose to define multi-container environments that allow running automated test cases in parallel. This leverages available resources to scale test execution and reduce overall time.
- Automate Image Building: Integrate Docker with CI/CD pipelines for automated image building after code changes. Use tags like git commit SHA to version images. This enables traceability and reproducibility.
- Limit Container Lifetime: Avoid long-running containers and tear down containers after each test run. This prevents wasting unused resources. Docker Compose helps manage short-lived containers.
- Leverage Docker Registries: Share test containers through registries like Docker Hub or self-hosted registries. This promotes reuse across teams and projects and synchronized container versions.
- Validate Across Infrastructure: Run tests across different infra – local, CI, and cloud – to validate portability. Containers should exhibit consistent behavior across environments.
- Traceability: Implement full traceability from code changes through image builds and test runs. Integrations with Git, CI, and Docker provide change tracking.
Conclusion
Containerizing Playwright tests with Docker provides a consistent, portable, and efficient way to execute automated browser testing. Leveraging Docker delivers significant advantages for test reliability, reproducibility, and environmental consistency.
By building a Docker image with Playwright and test dependencies preinstalled, we can produce a standardized container that bundles everything needed to run the tests. This container can then execute the Playwright tests exactly the same way regardless of the underlying host infrastructure.
Docker makes it easy to supply sensitive test data files and environment variables needed for Playwright scripts. Docker’s separation between containers and host filesystems offers a secure method to inject credentials, API keys, test data, etc, into the test runtime without hardcoding in scripts.
In addition, Docker Compose enables simple orchestration of the full test environment, including databases, proxies, mock servers, etc. Using a declarative Compose file, we can spin up all dependent services with the proper configuration to support our Playwright tests.
Overall, containerization provides portability, encapsulation, and reproducibility for browser testing. Tests running inside Docker containers exhibit consistent behavior across platforms and environments. Docker enables reliable Playwright testing, executed locally, on CI infrastructure, or across multiple cloud hosts.
The standardization and isolation of Docker combined with Playwright’s browser test automation delivers an optimized approach for functional testing web apps and sites. Containerized testing revolutionizes test efficiency, consistency, and scalability.